HTML5’s Drag and Drop Problem
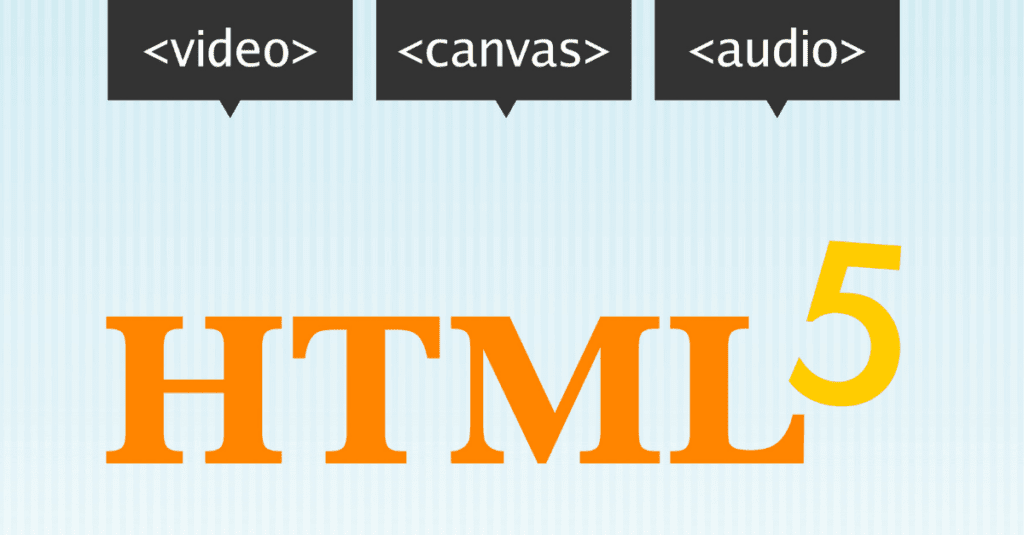
The HTML5 drag and drop specification originated from an effort to emulate behavior introduced in Internet Explorer 5 (IE5). Initially, this was a pragmatic choice, aiming to leverage IE support at a time when legacy browser compatibility was a significant concern.
However, the HTML5 drag and drop API, now integrated into most browsers and supporting cross-document operations, still presents challenges. It combines native and web behaviors in an uneasy mix and repurposes DOM methods like event.preventDefault()
for purposes that might seem conceptually confusing.
Yet, despite its integration across browsers and its ability to facilitate dragging in files and content from the desktop, using the native API for core interactions within an application might not be ideal.
Native API Limitations
DataTransfer Object The DataTransfer object, integral to the drag-and-drop API, allows data exchange between web and native environments. However, it imposes strict security policies that restrict mid-drag access to the data payload, hindering predictive feedback about potential drop actions. This limitation affects the ability to anticipate invalid drop actions, leading to less intuitive interfaces.
Several workarounds exist, such as manipulating MIME types to pass information or breaking encapsulation to introduce a shared application state. However, these approaches undermine the original purpose of using the DataTransfer object.
Visual Feedback The API’s capability to provide visual feedback in the form of a drag “ghost” falls short. The native generation of the ghost, usually a screenshot of the dragged element, lacks flexibility and doesn’t adequately reflect dynamic changes or user-generated content. Overriding this default behavior with custom images or canvases is possible but has limitations, especially when dealing with user-provided HTML.
Design Issues and Bugs
The HTML5 drag-and-drop API attempts to serve both as a translator between web and native behaviors and as a UI library for managing drag interactions. This hybrid functionality often leads to implementation bugs and inconsistencies across browsers.
Additionally, browser behavior during drag events—such as interface elements freezing or scrolling features becoming disabled—varies and may affect user experience. Inconsistencies in browser implementations, like missing properties or rendering bugs, further contribute to the challenges of using the native API.
Practical Approach
Given these limitations and challenges, leveraging the native drag-and-drop API solely for exchanging data with the operating system, such as importing files from the desktop, remains a practical use case.
Developers often resort to custom drag-and-drop libraries that complement native behaviors without replicating them entirely. These libraries focus on addressing specific limitations of the native API, offering more stability and clearer interactions.
Future Outlook
Improvements in libraries and ongoing bug filings for browser enhancements signify a continual evolution in drag-and-drop capabilities on the web. While drag and drop might not always be the optimal solution for designing interfaces, efforts to enhance both browser APIs and custom solutions contribute to its evolution as a reliable tool for direct manipulation on the web.
Cross-document communication using DOM methods has its complexities, requiring inventive approaches involving divs, DOM calculations, and document.elementFromPoint()
.
In essence, while the native HTML5 drag-and-drop API offers certain capabilities, its limitations prompt developers to seek alternative solutions tailored to their specific use cases, fostering a more adaptable and effective approach to drag-and-drop interactions on the web.
Further Reading
- HTML5 Drag and Drop in Opera – An updated look at compatibility notes across various browser implementations, offering insights into the history and current state of HTML5 drag and drop.
- The HTML5 Drag and Drop Disaster by QuirksMode – An opinionated piece from PPK, featuring discussions and viewpoints on HTML5 drag and drop, with insights from the past to present.
- Drag Operations at MDN – A comprehensive resource at MDN Web Docs, providing detailed information about drag behaviors, despite not being a direct tutorial.
- Reverse-Engineering IE5 Drag and Drop by Ian Hickson – Delve into the origins of HTML5 drag and drop through insights from the editor of the HTML5 spec, offering historical context.
- The HTML5 Drag and Drop Spec (Living Standard) – A direct link to the living standard specification for HTML5 drag and drop, offering technical details and guidelines for implementation.